Project: Tic-tac-toe#
import numpy as np
import matplotlib.pyplot as plt
X-figure#
N = 10
x = np.ones((N+2,N+2))
for i in range(1,N):
x[i,i] = 0
x[i, N-i] = 0
plt.imshow(x, 'gray')
plt.axis('off');
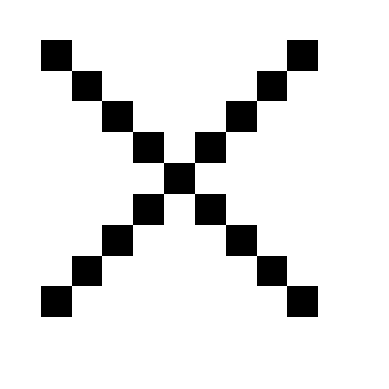
vx = np.vstack([x,x,x])
plt.imshow(vx, 'gray')
plt.axis('off');
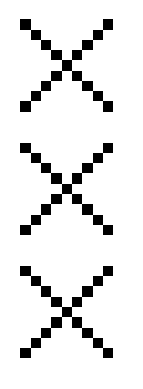
vx = np.vstack([x,x,x])
plt.imshow(np.hstack([vx, vx, vx]), 'gray')
plt.axis('off');
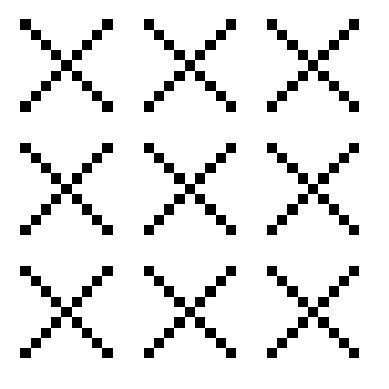
square-figure#
N = 10
s = np.ones((N+2,N+2))
for i in range(2,N-1):
s[2,i] = 0
s[N-2,i] = 0
s[i, 2] = 0
s[i, N-2] = 0
plt.imshow(s, 'gray')
plt.axis('off');
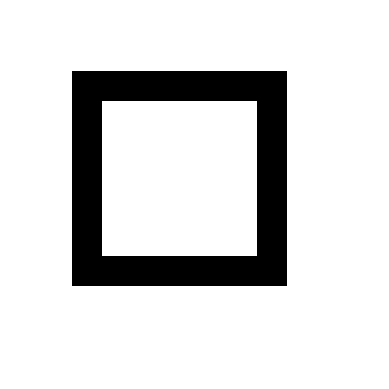
vs = np.vstack([s,s,s])
plt.imshow(np.hstack([vs, vs, vs]), 'gray')
plt.axis('off');
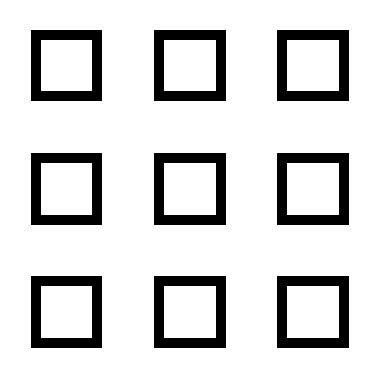
plt.figure(figsize=(5,5))
for i in range(4):
plt.plot([i,i], [0,3], c='r')
plt.plot([0,3], [i,i], c='r')
plt.xticks([0,1,2,3])
plt.yticks([0,1,2,3]);
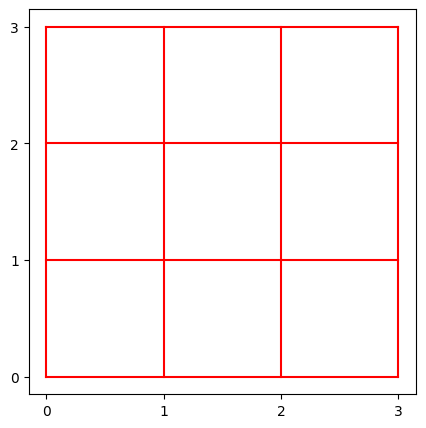
location = {}
n = 1
for i in range(3):
for j in range(3):
location[n] = (j+0.1, 2-i+0.05)
n += 1
location
{1: (0.1, 2.05),
2: (1.1, 2.05),
3: (2.1, 2.05),
4: (0.1, 1.05),
5: (1.1, 1.05),
6: (2.1, 1.05),
7: (0.1, 0.05),
8: (1.1, 0.05),
9: (2.1, 0.05)}
def field():
plt.figure(figsize=(5,5))
for i in range(4):
plt.plot([i,i], [0,3], c='r')
plt.plot([0,3], [i,i], c='r')
plt.xticks([0,1,2,3])
plt.yticks([0,1,2,3])
for n in range(1,10):
plt.text(location[n][0], location[n][1], str(n), fontsize=100, alpha=0.03)
field()
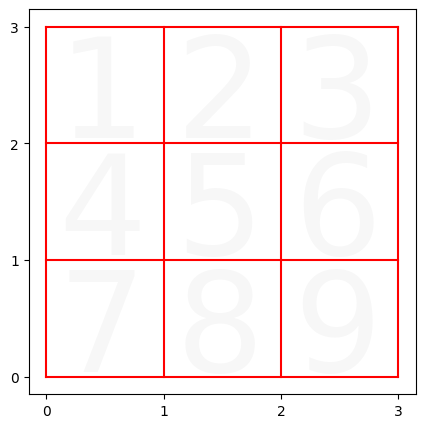
def end_game(show=list(range(1,10))):
if (show[0] == show[1] == show[2]): return True, [0,1,2]
if (show[3] == show[4] == show[5]): return True, [3,4,5]
if (show[6] == show[7] == show[8]): return True, [6,7,8]
if (show[0] == show[3] == show[6]): return True, [0,3,6]
if (show[1] == show[4] == show[7]): return True, [1,4,7]
if (show[2] == show[5] == show[8]): return True, [2,5,8]
if (show[0] == show[4] == show[8]): return True, [0,4,8]
if (show[2] == show[4] == show[6]): return True, [2,4,6]
return False
end_game(['O','X','O','O','X','O','O', 'O', 'X'])
(True, [0, 3, 6])
show = np.array(['O','X','O','O','X','O','O', 'O', 'X'])
len(set(show[[0,3]]))
1
case = [3,4,5]
x = [location[i+1][0]+0.2 for i in case]
y = [location[i+1][1] for i in case]
plt.plot(x,y, linewidth=50, alpha=0.3);
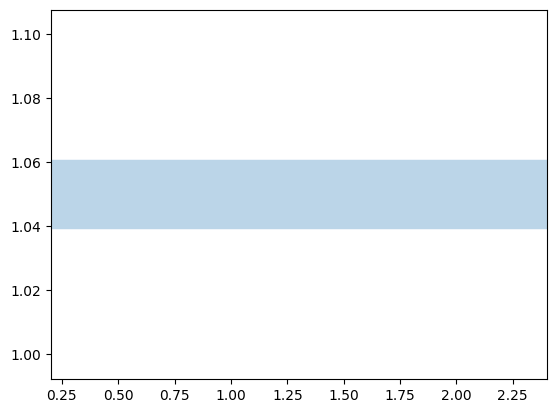
def end_game(show=list(range(1,10))):
finish_cases = [ [0,1,2],[3,4,5], [6,7,8], [0,3,6], [1,4,7], [2,5,8], [0,4,8], [2,4,6] ]
show_array = np.array(show)
for case in finish_cases:
if len(set(show_array[case])) == 1:
x = [location[i+1][0]+0.45 for i in case]
y = [location[i+1][1]+0.45 for i in case]
return True, [x,y]
break
return False, ''
end_game(['O','O','O',4,'X','O','O', 'O', 'X'])
(True, [[0.55, 1.55, 2.5500000000000003], [2.5, 2.5, 2.5]])
def game(show=list(range(1,10))):
plt.figure(figsize=(5,5))
for i in range(4):
plt.plot([i,i], [0,3], c='r')
plt.plot([0,3], [i,i], c='r')
for n in range(9):
if show[n] == 'X':
plt.text(location[n+1][0], location[n+1][1], 'X', fontsize=100)
elif show[n] == 'O':
plt.text(location[n+1][0], location[n+1][1], 'O', fontsize=100)
else:
plt.text(location[n+1][0], location[n+1][1], show[n], fontsize=100, alpha=0.03)
plt.xticks([0,1,2,3])
plt.yticks([0,1,2,3])
game()
game(['O',2,'O',4,5,6,7,'X',9])
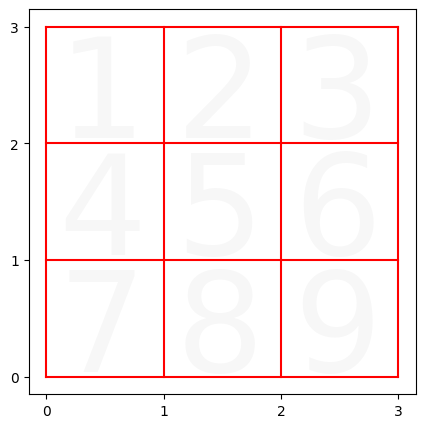
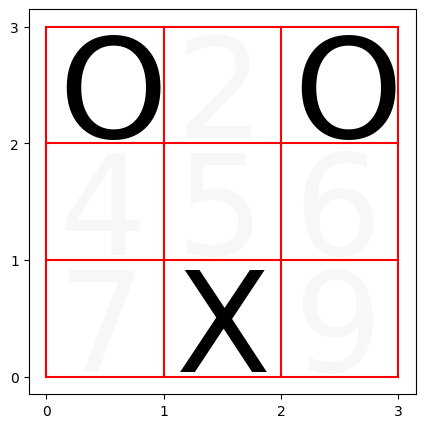
# available = list(range(1,10))
# current = list(range(1,10))
# import random
# import time
# game(current)
# while len(available)>2:
# user = int(input('Choose a number: '))
# if user in available:
# available.remove(user)
# current[user-1] = 'X'
# # plt.close()
# game(current)
# plt.show();
# if end_game(current)[0]:
# print('Game is over! Winner is X')
# # plt.plot(end_game(current)[1][0], end_game(current)[1][1], linewidth=50, alpha=0.3);
# break
# computer = random.choice(available)
# print(f'Computer choice: {computer}')
# available.remove(computer)
# current[computer-1] = 'O'
# # plt.close()
# game(current)
# plt.show();
# if end_game(current)[0]:
# print('Game is over! Winner is O')
# # plt.plot(end_game(current)[1][0], end_game(current)[1][1], linewidth=50, alpha=0.3);
# break
# time.sleep(1)
# else:
# print('Choose an available number!')
# # plt.close()
# game(current)
# plt.plot(end_game(current)[1][0], end_game(current)[1][1], linewidth=120, alpha=0.3, color='orange');
# plt.show()
# if not end_game(current):
# current[available[0]-1] = 'X'
# # plt.close()
# game(current)
# plt.show();
available = list(range(1,10))
current = list(range(1,10))
import random
import time
game(current)
plt.show()
print('Welcome to Tic-Tac-Toe Game!!!!\nGood Luck!')
time.sleep(2)
while len(available)>2:
user = int(input('Choose a number: '))
if user in available:
available.remove(user)
current[user-1] = 'X'
game(current)
plt.show()
if end_game(current)[0]:
print('Game is over! Winner is X')
break
clear_output(wait = True)
time.sleep(2)
computer = random.choice(available)
print(f'Computer choice: {computer}')
available.remove(computer)
current[computer-1] = 'O'
game(current)
plt.show()
clear_output(wait = True)
time.sleep(2)
if end_game(current)[0]:
print('Game is over! Winner is O')
break
else:
print('Choose an available number!')
clear_output(wait = True)
game(current)
plt.plot(end_game(current)[1][0], end_game(current)[1][1], alpha=0.3, linewidth=80, color='orange');
plt.show()
if not end_game(current):
current[available[0]-1] = 'X'
game(current)
plt.show();
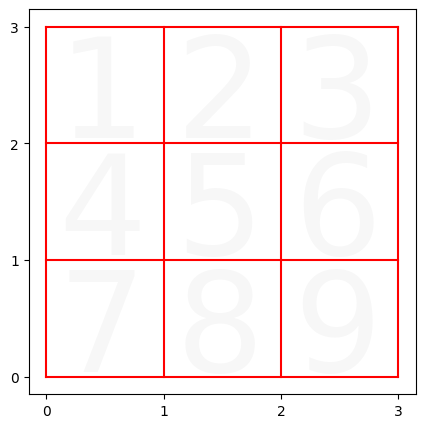
Welcome to Tic-Tac-Toe Game!!!!
Good Luck!
---------------------------------------------------------------------------
StdinNotImplementedError Traceback (most recent call last)
Cell In[16], line 12
9 time.sleep(2)
11 while len(available)>2:
---> 12 user = int(input('Choose a number: '))
13 if user in available:
14 available.remove(user)
File ~/anaconda3/lib/python3.11/site-packages/ipykernel/kernelbase.py:1201, in Kernel.raw_input(self, prompt)
1199 if not self._allow_stdin:
1200 msg = "raw_input was called, but this frontend does not support input requests."
-> 1201 raise StdinNotImplementedError(msg)
1202 return self._input_request(
1203 str(prompt),
1204 self._parent_ident["shell"],
1205 self.get_parent("shell"),
1206 password=False,
1207 )
StdinNotImplementedError: raw_input was called, but this frontend does not support input requests.